How to add a dynamic field in a custom section
Add the following code in your theme’s functions.php file. The custom_section from the hook before_wpccf_custom_section_form_fields or after_wpccf_custom_section_form_fields must be replaced with the slug of the custom section you have added. Below is the steps on how to know the slug of your custom section.
- Right click the custom section and click the Inspect Element.
- You will find the ID of the section from the elementor. That is the slug of your custom section. Find in below screenshot.
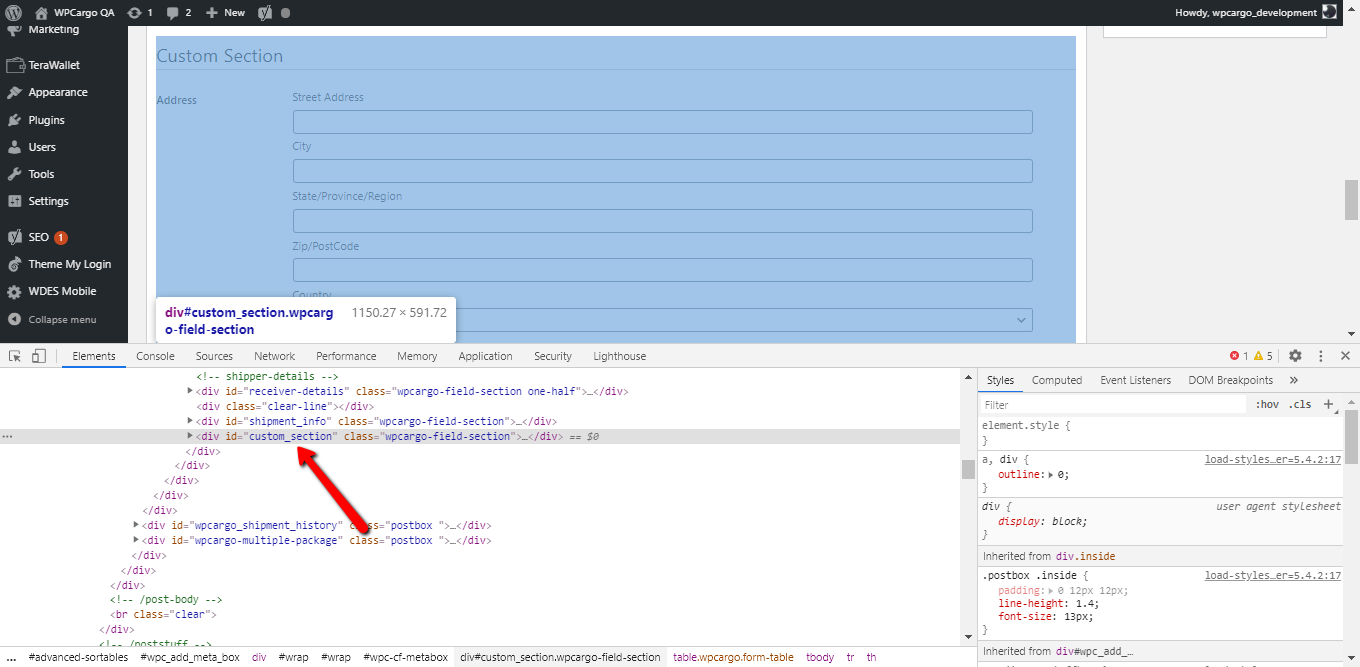
This adds the dynamic field at the top of the custom section
add_action( 'before_wpccf_custom_section_form_fields', 'custom_shipment_info_additional_field' ); function custom_shipment_info_additional_field( $shipment_id ){ global $wpcargo; if( !$shipment_id ){ $shipment_id = 0; } $customer = get_post_meta( $shipment_id, 'customer_fields', true ); $args = array( 'role__in' => array('wpcargo_client'), 'orderby' => 'display_name', 'order' => 'ASC' ); $users = array(); if( !empty( get_users( $args ) ) ){ foreach ( get_users( $args ) as $user ) { $users[$user->ID] = $wpcargo->user_fullname( $user->ID ); } } ?> <div id="form-client-list" class="wpcargo-field-section"> <h1>Additional Information</h1> <table class="wpcargo form-table"> <tbody><tr> <th><label>Customer Name</label></th> <td> <select name="customer_fields" class="mdb-select mt-0 form-control browser-default" id="customer_fields" required=""> <option value=""><?php _e('-- Select Customer --','wpcargo-frontend-manager'); ?></option> <?php if( !empty( $users ) ): ?> <?php foreach( $users as $key => $value ): ?> <option value="<?php echo $key; ?>" <?php selected( $customer, $key ); ?>><?php echo $value; ?></option> <?php endforeach; ?> <?php endif; ?> </select> </td> </tr> </table> </div> <?php }
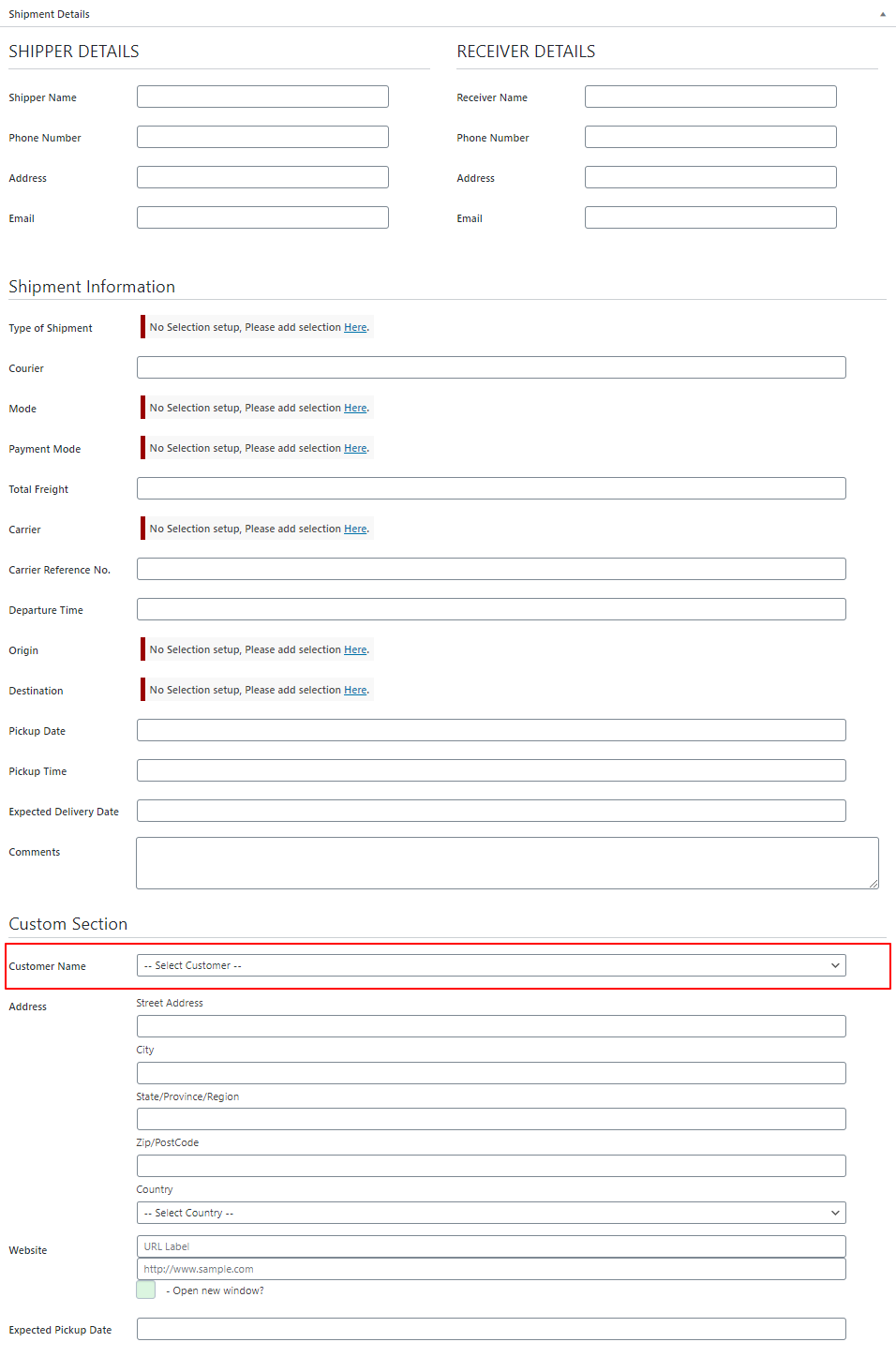
This adds the dynamic field at the bottom of the custom section
add_action( 'after_wpccf_custom_section_form_fields', 'custom_shipment_info_additional_field' ); function custom_shipment_info_additional_field( $shipment_id ){ global $wpcargo; if( !$shipment_id ){ $shipment_id = 0; } $customer = get_post_meta( $shipment_id, 'customer_fields', true ); $args = array( 'role__in' => array('wpcargo_client'), 'orderby' => 'display_name', 'order' => 'ASC' ); $users = array(); if( !empty( get_users( $args ) ) ){ foreach ( get_users( $args ) as $user ) { $users[$user->ID] = $wpcargo->user_fullname( $user->ID ); } } ?> <div id="form-client-list" class="wpcargo-field-section"> <h1>Additional Information</h1> <table class="wpcargo form-table"> <tbody><tr> <th><label>Customer Name</label></th> <td> <select name="customer_fields" class="mdb-select mt-0 form-control browser-default" id="customer_fields" required=""> <option value=""><?php _e('-- Select Customer --','wpcargo-frontend-manager'); ?></option> <?php if( !empty( $users ) ): ?> <?php foreach( $users as $key => $value ): ?> <option value="<?php echo $key; ?>" <?php selected( $customer, $key ); ?>><?php echo $value; ?></option> <?php endforeach; ?> <?php endif; ?> </select> </td> </tr> </table> </div> <?php }
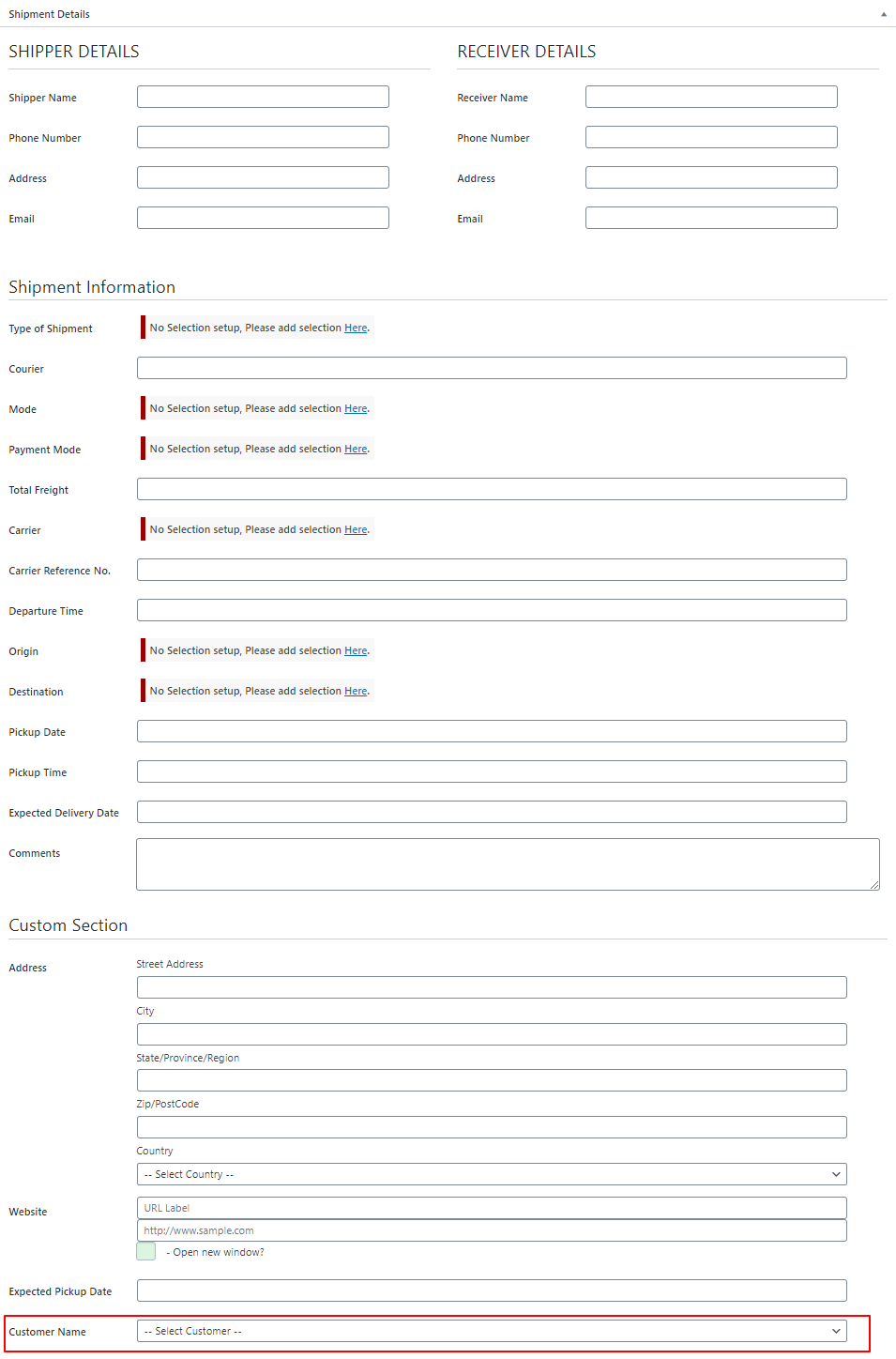
Following code works as saving of the data of your custom field
add_action( 'save_post', 'save_custom_shipment_info_additional_field' ); function save_custom_shipment_info_additional_field( $shipment_id ){ update_post_meta( $shipment_id, 'customer_fields', $_POST['customer_fields'] ); }